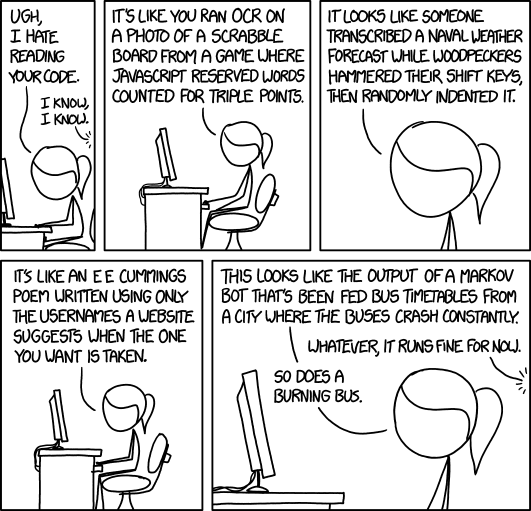
3/19/2019
Back to last week… Good Names are…
Question: Functions can be used to do which of the following:
What are some things that make a good function?
get_em = function(orders){ flagged_orders = list() for (order in orders){ if (is_flagged_order(order)){ flagged_orders = append(flagged_orders, order) } } return(flagged_orders) }
What are some better names for this function?
extract_flagged_orders = function(orders){ flagged_orders = list() for (order in orders){ if (is_flagged_order(order)){ flagged_orders = append(flagged_orders, order) } } return(flagged_orders) }
The single responsibility principle is a computer programming principle that states that every module, class, or function should have responsibility over a single part of the functionality provided by the software, and that responsibility should be entirely encapsulated by the class.
a.k.a. Curly’s Law
a.k.a. Don’t let your code turn into a rat’s nest
Fewer inputs require less brain-power to understand.
# Zero-Inputs (niladic function) data = get_data() # One Input (monadic function) data = get_data(path = "../data/test.csv") # Two Inputs (dyadic function) data = get_data(path = "../data/test.csv", is_train = FALSE) # Three Inputs (triadic function) data = get_data(path = "..data/test.csv", is_train = FALSE, scale = FALSE)
get_data
?def get_transformed_credit_card_data(path_to_data, is_training): """Get and transform credit card data into f""" data = get_credit_card_data(path_to_data) data = handle_duplicate_records(data) data = transform_categoical_features(data) data = transform_bill_and_pay_amount(data) data = generate_total_months_delinqient(data) if is_training: data = transform_target_variable(data) return data
If code
== writing
, then functions can be framed as structured paragraphs. They have topic sentences, supporting details, and a conclusion.
Sarcasm is a weird way of pronouncing depression (source: https://t.co/BsPr9oY3Se ) #reddit #dev #meme pic.twitter.com/K1Zx2ZQ3TC
— Code Memes (@CodeDoesMeme) November 4, 2018
In computer science, an operation, function or expression is said to have a side effect if it modifies some state variable value(s) outside its local environment, that is to say has an observable effect besides returning a value (the main effect) to the invoker of the operation.
Common Side Effects:
def calculate_sum(a, b): print(a) pickle.dump(b, open("second_argument.p", "wb" )) sum_vals = a + b return(sum_vals) total = calculate_sum(1, 2) print(total) print(sum_vals)
Good functions do the following…
Description: Find a partner and exchange a block of code you have written. 50-100 lines. Try and refactor the code based on our discussion while maintaining functionality. For the first 10 minutes try not to ask any questions, and allow the code to “speak” for itself.
Time: 20 Minutes Total